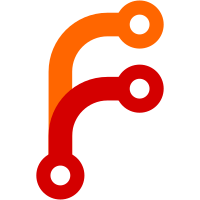
- Added standard .gitattributes file for Zig projects. - Reworked build.zig a little, hopefully it's a bit clearer. Also, now zig build will run all steps. - outer: while in examples was redundant since there's only one loop to break from. switch expressions don't allow breaking from them, so breaking is only for loops, i.e. while and for. - When returning a struct instance from a function, the compiler infers the return type from function signature, so instead of return MyType{...}; , it's more idiomatic to write return .{...};. - Logging adds a new line by default, so you don't usually need to write \n like here: log.debug("event: {}\r\n", .{event});.
58 lines
1.3 KiB
Zig
58 lines
1.3 KiB
Zig
const Image = @import("Image.zig");
|
|
|
|
char: Character = .{},
|
|
style: Style = .{},
|
|
link: Hyperlink = .{},
|
|
image: ?Image.Placement = null,
|
|
|
|
/// Segment is a contiguous run of text that has a constant style
|
|
pub const Segment = struct {
|
|
text: []const u8,
|
|
style: Style = .{},
|
|
link: Hyperlink = .{},
|
|
};
|
|
|
|
pub const Character = struct {
|
|
grapheme: []const u8 = " ",
|
|
/// width should only be provided when the application is sure the terminal
|
|
/// will measure the same width. This can be ensure by using the gwidth method
|
|
/// included in libvaxis. If width is 0, libvaxis will measure the glyph at
|
|
/// render time
|
|
width: usize = 1,
|
|
};
|
|
|
|
pub const Hyperlink = struct {
|
|
uri: []const u8 = "",
|
|
/// ie "id=app-1234"
|
|
params: []const u8 = "",
|
|
};
|
|
|
|
pub const Style = struct {
|
|
pub const Underline = enum {
|
|
off,
|
|
single,
|
|
double,
|
|
curly,
|
|
dotted,
|
|
dashed,
|
|
};
|
|
|
|
fg: Color = .default,
|
|
bg: Color = .default,
|
|
ul: Color = .default,
|
|
ul_style: Underline = .off,
|
|
|
|
bold: bool = false,
|
|
dim: bool = false,
|
|
italic: bool = false,
|
|
blink: bool = false,
|
|
reverse: bool = false,
|
|
invisible: bool = false,
|
|
strikethrough: bool = false,
|
|
};
|
|
|
|
pub const Color = union(enum) {
|
|
default,
|
|
index: u8,
|
|
rgb: [3]u8,
|
|
};
|