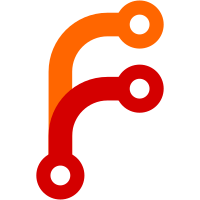
the man page for macOS `poll(2)` says, under "bugs", that polling devices does not work, and indeed, although the current implementation based on poll(2) does produce output, it does not appear to actually be polling for this reason; instead the wait is blocking on the `read` call, causing the macOS user to need to input another character to exit. this change introduces a wrapper over macOS's implementation of `select`, modeled after `std.io.poll` as `select.zig`. it is a compile error to use `select.zig` when `builtin.os.tag.isDarwin()` is false. a lightly altered version of `Tty.zig` accompanies this change. it's certainly possible to incorporate the two into one file; i didn't just to leave the other one in its original state. with this change, writing to the pipe correctly exits. additionally, on macOS, `Thread.setName` must be called from the thread whose name you wish to set, so I have just commented out that line.
77 lines
2.3 KiB
Zig
77 lines
2.3 KiB
Zig
const std = @import("std");
|
|
|
|
pub fn build(b: *std.Build) void {
|
|
const target = b.standardTargetOptions(.{});
|
|
const optimize = b.standardOptimizeOption(.{});
|
|
const root_source_file = std.Build.LazyPath.relative("src/main.zig");
|
|
|
|
// Dependencies
|
|
const ziglyph_dep = b.dependency("ziglyph", .{
|
|
.optimize = optimize,
|
|
.target = target,
|
|
});
|
|
const zigimg_dep = b.dependency("zigimg", .{
|
|
.optimize = optimize,
|
|
.target = target,
|
|
});
|
|
|
|
// Module
|
|
const vaxis_mod = b.addModule("vaxis", .{
|
|
.root_source_file = root_source_file,
|
|
.target = target,
|
|
.optimize = optimize,
|
|
});
|
|
vaxis_mod.addImport("ziglyph", ziglyph_dep.module("ziglyph"));
|
|
vaxis_mod.addImport("zigimg", zigimg_dep.module("zigimg"));
|
|
|
|
// Examples
|
|
const example_step = b.step("example", "Run examples");
|
|
|
|
const example = b.addExecutable(.{
|
|
.name = "vaxis_pathological_example",
|
|
// Change this to the example you want to use!
|
|
.root_source_file = std.Build.LazyPath.relative("examples/text_input.zig"),
|
|
.target = target,
|
|
.optimize = optimize,
|
|
});
|
|
example.root_module.addImport("vaxis", vaxis_mod);
|
|
|
|
const example_run = b.addRunArtifact(example);
|
|
example_step.dependOn(&example_run.step);
|
|
|
|
// Tests
|
|
const tests_step = b.step("test", "Run tests");
|
|
|
|
const tests = b.addTest(.{
|
|
.root_source_file = .{ .path = "src/Tty-macos.zig" },
|
|
.target = target,
|
|
.optimize = optimize,
|
|
});
|
|
tests.root_module.addImport("ziglyph", ziglyph_dep.module("ziglyph"));
|
|
tests.root_module.addImport("zigimg", zigimg_dep.module("zigimg"));
|
|
|
|
const tests_run = b.addRunArtifact(tests);
|
|
b.installArtifact(tests);
|
|
tests_step.dependOn(&tests_run.step);
|
|
|
|
// Lints
|
|
const lints_step = b.step("lint", "Run lints");
|
|
|
|
const lints = b.addFmt(.{
|
|
.paths = &.{ "src", "build.zig" },
|
|
.check = true,
|
|
});
|
|
|
|
lints_step.dependOn(&lints.step);
|
|
b.default_step.dependOn(lints_step);
|
|
|
|
// Docs
|
|
const vaxis_docs = tests;
|
|
const build_docs = b.addInstallDirectory(.{
|
|
.source_dir = vaxis_docs.getEmittedDocs(),
|
|
.install_dir = .prefix,
|
|
.install_subdir = "docs",
|
|
});
|
|
const build_docs_step = b.step("docs", "Build the vaxis library docs");
|
|
build_docs_step.dependOn(&build_docs.step);
|
|
}
|